Introducing the Together Embeddings endpoint — Higher accuracy, longer context, and lower cost
Today, we are excited to release the Together Embeddings endpoint! Some of the highlights are:
- 8 leading embedding models – including models that outperform OpenAI’s ada-002 and Cohere’s Embed-v3 in MTEB and LoCo Benchmarks
- State-of-the-art long context M2-Retrieval models up to 32k context length
- Up to 4x cheaper than other popular platforms
- Integrations with MongoDB, LangChain, and LlamaIndex for RAG
- A fully OpenAI compatible API to make migrating easy
Text embeddings provide a measure of the similarity or relatedness of given text queries. Therefore, embeddings are used for various applications including clustering, semantic search, and classification. Additionally, there has been an increasing interest in retrieval augmented generation, or RAG, which aims to overcome limitations of generative AI models, such as a lack of knowledge from unseen data, by finding relevant information from a given knowledge base through embeddings and providing the information to a generative model.

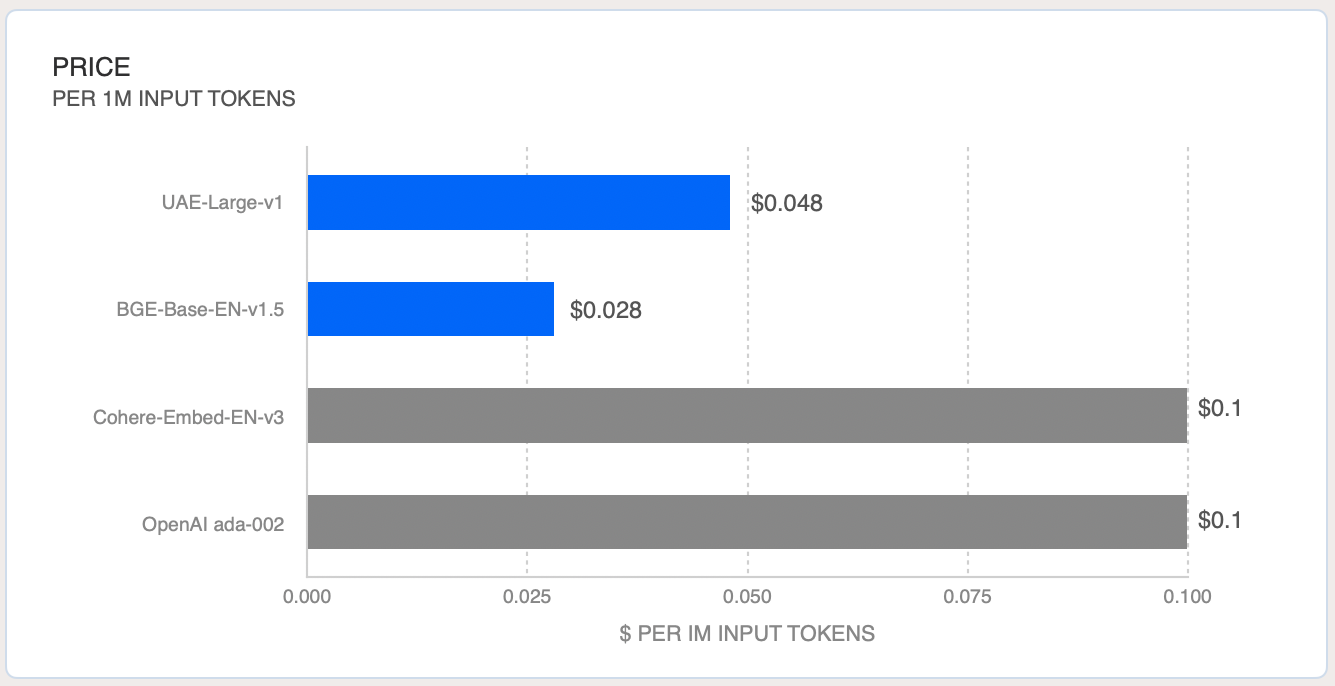
Together Embeddings endpoint is available today with 8 open source embeddings models, including top models from the MTEB leaderboard (Massive Text Embedding Benchmark), such as UAE-Large-v1 and BGE models, and the newly released M2-BERT retrieval models for long context (2k, 8k, 32k). See the full list of embeddings models here.
These models achieve state-of-the-art performance in MTEB showing comparable or even better accuracies than closed models. Additionally, M2-BERT retrieval models significantly outperform other closed models in long context retrieval tasks. This means you can now generate embeddings for long documents without splitting them into many short chunks while containing more meaningful contexts in the embeddings. You can also access these powerful models at very competitive prices (up to 4x cheaper) as seen in the pricing graph below.
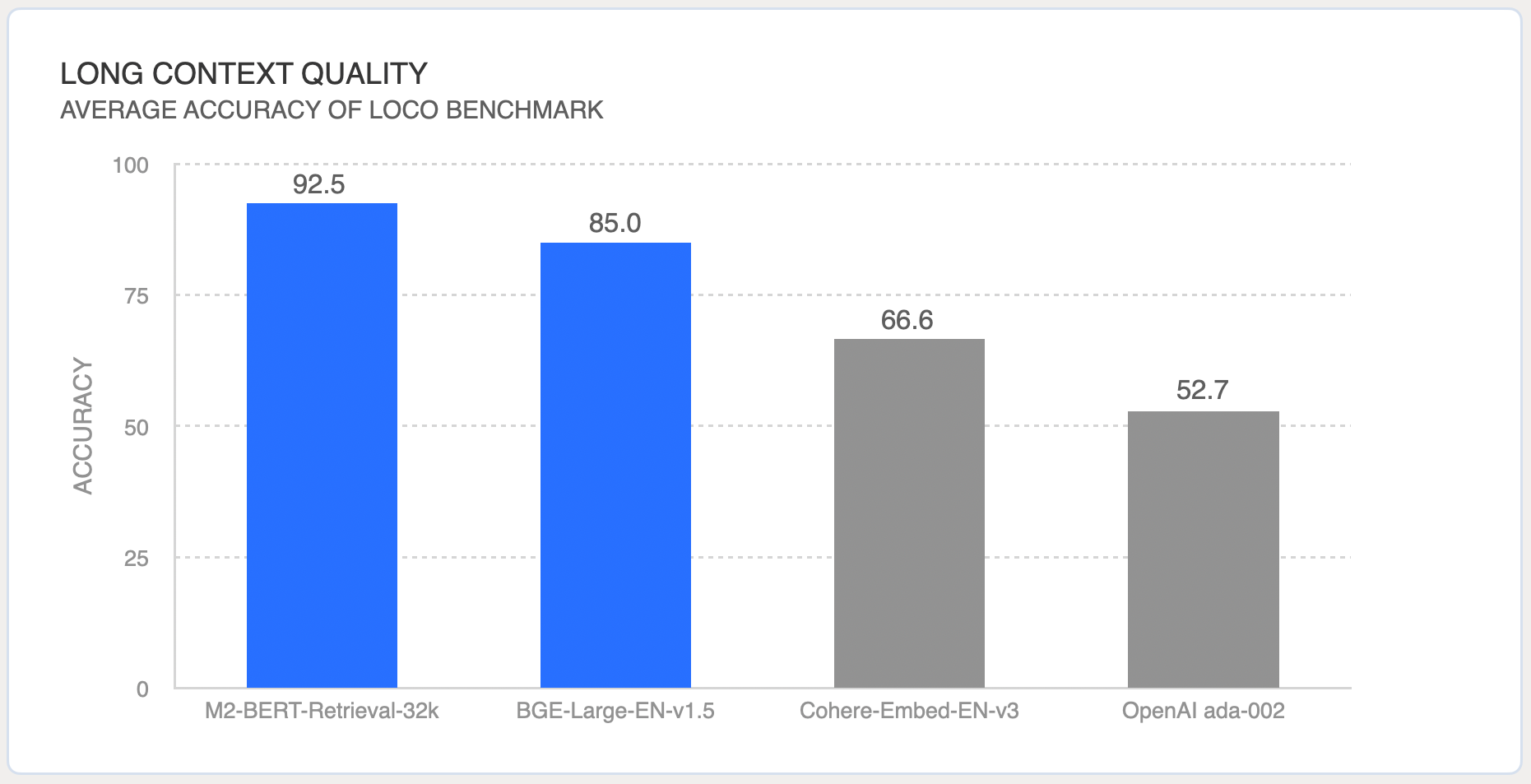

Similar to our Inference endpoint, we will continue to add top open source embeddings models to our platform, so that our developers can continue to build successful AI applications! Request new embeddings models by filling out this form.
OpenAI API Compatibility
The Together Embeddings endpoint has full OpenAI compatibility so if you have already built your applications following the OpenAI API you can easily switch it out:
Building RAG with Together Embeddings
One of the most popular use cases of embeddings models is building a Retrieval Augmented Generation (RAG) system. You can now build RAG using Together API and popular vector databases such as MongoDB, Pinecone, and Chroma or by leveraging frameworks such as LangChain and LlamaIndex. Check out the following blogs where we give you a deep-dive tutorial on how to build RAG using Together with MongoDB, using Together with LangChain, or using Together with LlamaIndex. You can learn more in our documentation.
Data Visualization Example
Embeddings can be used in various applications. In this section, we will take a look at how to use the Together Embeddings endpoint to visualize sample data from various domains of RedPajama-v1 Sample. The following code snippet shows an example of how to use one of the listed models, M2-BERT-Retrieval-8K (API string: togethercomputer/m2-bert-80M-8k-retrieval), for data visualization.
First, define functions to extract samples from a data file and generate embeddings using the Together API.
The dimension of embedding vectors is usually too large to easily visualize. For M2-BERT-Retrieval-8K, the embedding dimension is 768. To plot each data example in 2D, we will use tSNE to transform the embedding vectors.
In the main script, run the following to display the plot:
As expected, texts from each data source are somewhat closely located. We can also see the similarities between github and stackexchange, and between book and c4, while github and book are less related.
.webp)
Get Started with Together API
Data visualization is just one of many use cases where embeddings can be used. Explore what you can do using embeddings by accessing all the models on our platform through the Together API. For more information, see our documentation.
- Lower
Cost20% - faster
training4x - network
compression117x
Q: Should I use the RedPajama-V2 Dataset out of the box?
RedPajama-V2 is conceptualized as a pool of data that serves as a foundation for creating high quality datasets. The dataset is thus not intended to be used out of the box and, depending on the application, data should be filtered out using the quality signals that accompany the data. With this dataset, we take the view that the optimal filtering of data is dependent on the intended use. Our goal is to provide all the signals and tooling that enables this.